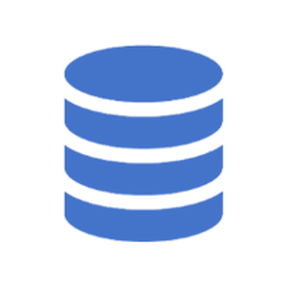
1. SQL Indexes
An index in SQLis a data structure that improves query performance by allowing MySQL to quickly locate data without scanning each row in a table. Indexes are especially useful for speeding up queries that use WHERE
clauses, JOINs
, and ORDER BY
conditions.
Types of Indexes:
- Single-column index: Created on one column, making lookups on that column faster.
- Composite (Multi-column) index: Created on multiple columns, optimized for queries that filter by a combination of these columns.
2. Memory Impact of Indexes
Storage Requirement: Indexes take up additional space on disk and in memory because they store pointers to rows along with indexed column values.
Memory Allocation:
- Indexes are loaded into memory (RAM) to allow quick access, impacting the buffer pool in MySQL.
- Write operations (like
INSERT
,UPDATE
, andDELETE
) may temporarily increase memory usage, as updating indexes requires additional processing.
3. Speed Impact of Indexes
Read Performance: With an index, MySQL can locate rows faster by using the index structure, skipping the need for a full table scan.
Write Performance: Although indexes speed up read operations, they can slow down writes because every change to the table also updates the index.
Checking Index Usage: You can use the EXPLAIN
command to confirm if a query is utilizing an index, which shows details of MySQL's query execution plan.
4. Heap Files
A heap file (or heap-organized table) is an unordered collection of rows. Data is stored in no particular order, so retrieving specific rows requires scanning each row unless an index is present.
Storage: Heap files are efficient for inserts, as new rows are appended to the end, making them fast for INSERT
operations.
5. Index and Heap File Interaction
Speeding Up Access: Adding an index to a heap file allows MySQL to locate rows quickly without scanning the entire file, which is beneficial for large tables.
Read vs. Write Performance:
- Read: Indexes help avoid full scans, which is critical for large tables stored as heap files.
- Write: Each
INSERT
,UPDATE
, orDELETE
requires the index to be updated, impacting write speed due to the need to keep indexes synchronized with the heap file.
Practical Usage
Creating an Index: Use the following SQL command to create a single-column index:
CREATE INDEX index_name ON table_name(column_name);
Composite Indexes: Create a composite index on multiple columns to optimize queries filtering by these columns together:
CREATE INDEX idx_multi ON table_name (col1, col2);
Removing an Index: If an index is no longer needed, it can be removed using:
DROP INDEX index_name ON table_name;
Checking Index Usage: Use the EXPLAIN command to see if a query is using an index:
EXPLAIN SELECT * FROM table_name WHERE indexed_column = 'value';
Summary
Indexes and heap files are essential concepts in database management:
- Indexes help MySQL quickly locate data, improving query performance but adding memory and processing overhead.
- Heap files store data without order, which can be beneficial for inserts but slow for queries without indexes.
- Combining indexes with heap files allows MySQL to perform efficiently even with large tables.
Using indexes effectively involves balancing memory, speed, and storage considerations for both read and write operations.